Bash - Test whether a file or folder exists
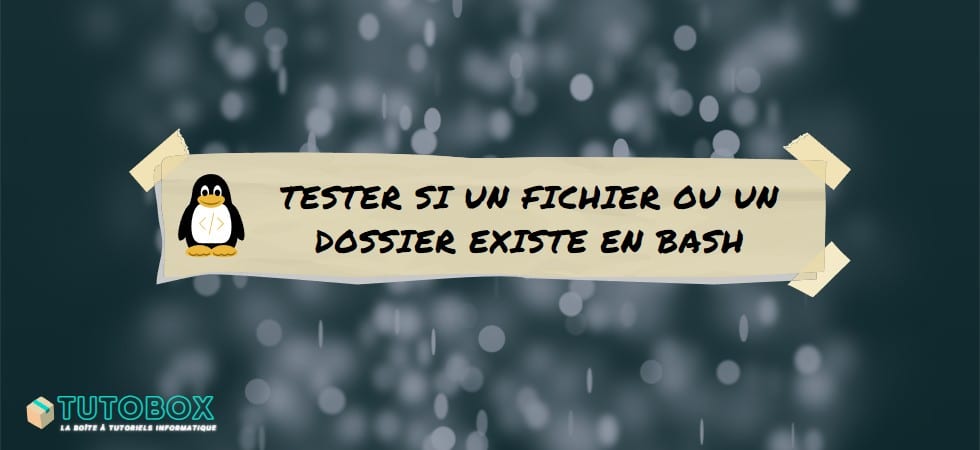
What's in the box?
Are you writing a Bash script and need to test whether a file or folder exists on your machine? Then this Linux-oriented article should appeal to you, because that's exactly what we're going to see!
On the same theme, you can read this article, but also browse the site! 😉
1. Test whether a file exists in Bash
To begin with, we're going to focus on files, since we'll be looking to check whether a file exists or not. For this, an "if" condition will enable us to perform this test quite easily.
In this example, we test the existence of the " /home/tutobox/my-file.txt" by storing this path in the FILE variable. Then, with an "if" condition and the "-f" condition, you can perform an action only if the file exists. Here, we write in the console "The file exists ()".
Here is the final result:
#!/bin/bash
FILE=/home/tutobox/my-file.txt
if [ -f "$FICHIER" ]; then
echo "The file exists ($FICHIER)"
fi
A pictorial result (based on other paths):

Rather than displaying a simple sentence, you could send an e-mail notification when the file is found (for example, to check for the presence of a backup file).
#!/bin/bash
FILE=/home/tutobox/my-file.txt
if [ -f "$FICHIER" ]; then
echo "Backup successful!" | mail -s "OK - Backup successful" destinataire@tutobox.fr -aFrom:expediteur@domaine.fr
fi
This involves configuring an agent on the local machine to send e-mails. Examples include MSMTP and Postfix.
To go even further, you can add an "else" condition for act if file does not exist. For example, if the file doesn't exist, you can create it with the "touch" command. Here again, the FILE variable is used. The result is :
#!/bin/bash
FILE=/home/tutobox/my-file.txt
if [ -f "$FICHIER" ]; then
echo "The file exists ($FICHIER)"
else
echo "The file does not exist ($FICHIER). It will be created".
touch $FICHIER
fi
For your information, you could also use the "test" command explicitly. The syntax would then be :
#!/bin/bash
FILE=/home/tutobox/my-file.txt
if test -f "$FICHIER"; then
echo "The file exists ($FICHIER)"
fi
Of course, this piece of code has to be written in a ".sh" file with the nano text editor (or another like vi), for example "file.sh", on which you'll have to assign execution rights before you can run it:
chmod +x file.sh
Then, to execute it :
./file.sh
2. Test whether a folder exists in Bash
To test whether a file exists or notWe can repeat the previous example, except that we're going to adjust the condition! Instead of using the "-f" condition for files, we'll use the "-d" condition for folders. For the rest, the principle remains the same!
In the script below, the FILE variable is used to determine the full path to the folder to be checked. Then, an "if/else" condition is used to indicate the action to be taken if it exists, and the action to be taken if it doesn't exist. If it doesn't exist, it will be created with mkdir. In both cases, an informative message is written to the Linux shell:
#!/bin/bash
DOSSIER=/home/tutobox/dossier
if [ -d "$DOSSIER" ]; then
echo "The folder exists ($DOSSIER)"
else
echo "The folder does not exist ($DOSSIER). It will be created".
mkdir $DOSSIER
fi
Here again, you need to create the script. For example, the "dossier.sh" script for this case:
nano dossier.sh
Include the above code in the script file "dossier.sh", adapting the path of the folder to be checked, and possibly adding other actions depending on the purpose of your script. Save the file.
Add execution rights to the "dossier.sh" file, otherwise we won't be able to run it on the local machine:
chmod +x dossier.sh
Then run this script to test it:
./folder.sh
3. Test if a file does not exist in Bash
You can also try to do the opposite and perform an action if the file (or folder) doesn't exist. In this case, you need to invert the condition with the "!" character. Here's an example based on what we've seen above:
#!/bin/bash
FILE=/home/tutobox/my-file.txt
if [ ! -f "$FICHIER" ]; then
echo "The file does not exist ($FICHIER)"
fi
It comes down to one character! 😉
4. Conclusion
Thanks to this article from the Computer Tutorials box, you'll be able to check whether a file or folder exists, or doesn't exist, in a Bash script under Linux!